Computes the maximum along segments of a tensor.
Read [the section on segmentation](https://tensorflow.org/api_docs/python/tf/math#Segmentation) for an explanation of segments.
Computes a tensor such that \\(output_i = \max_j(data_j)\\) where `max` is over `j` such that `segment_ids[j] == i`.
If the max is empty for a given segment ID `i`, `output[i] = 0`.
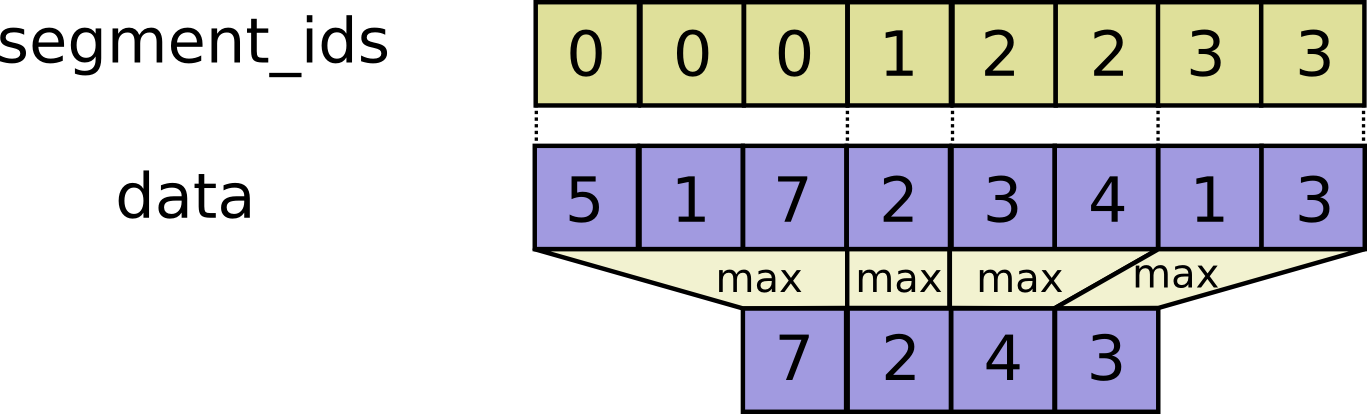
For example:
c = tf.constant([[1,2,3,4], [4, 3, 2, 1], [5,6,7,8]])
tf.segment_max(c, tf.constant([0, 0, 1]))
# ==> [[4, 3, 3, 4],
# [5, 6, 7, 8]]
Constants
String | OP_NAME | The name of this op, as known by TensorFlow core engine |
Public Methods
Output<T> |
asOutput()
Returns the symbolic handle of the tensor.
|
static <T extends TNumber> SegmentMax<T> | |
Output<T> |
output()
Has same shape as data, except for dimension 0 which
has size `k`, the number of segments.
|
Inherited Methods
Constants
public static final String OP_NAME
The name of this op, as known by TensorFlow core engine
Public Methods
public Output<T> asOutput ()
Returns the symbolic handle of the tensor.
Inputs to TensorFlow operations are outputs of another TensorFlow operation. This method is used to obtain a symbolic handle that represents the computation of the input.
public static SegmentMax<T> create (Scope scope, Operand<T> data, Operand<? extends TNumber> segmentIds)
Factory method to create a class wrapping a new SegmentMax operation.
Parameters
scope | current scope |
---|---|
segmentIds | A 1-D tensor whose size is equal to the size of `data`'s first dimension. Values should be sorted and can be repeated. |
Returns
- a new instance of SegmentMax
public Output<T> output ()
Has same shape as data, except for dimension 0 which has size `k`, the number of segments.